Online Platform
Kashier provides you with 3 Payment Integration methods for your online platform. You can integrate using prebuilt checkout forms or direct APIs.

i-frame
No redirection
Prebuilt
Fast & easy integration
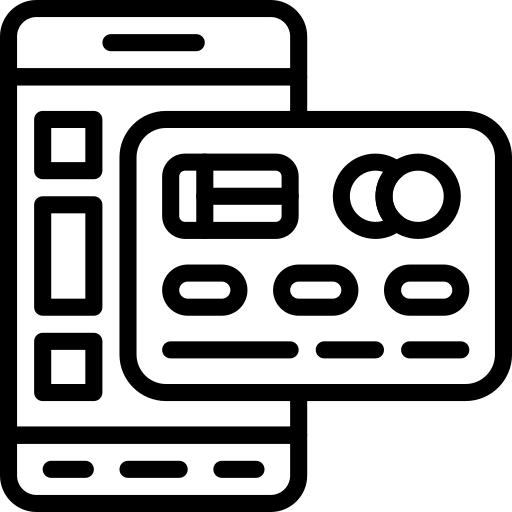
Embedded form (soon)
No redirection
Customizable
Fast & easy integration

Hosted Payment Page
Redirection
Prebuilt
Fast & easy integration
i-frame
The i-frame is a checkout popup window which opens on-top of your payment page. This method is recommended if you prefer a fast and easy way of integration without any customizations.
The i-frame is a prebuilt payment form, which is easy to integrate in only 4 steps. You can test the form by generating a Test API Key.
1. Setup your Kashier account
Sign up to create a Kashier account
2. Generate an API Key
You can generate multiple i-frame API Keys as per your acceptance channels.
- Login to Kashier platform
- Navigate to Integrate now page
- Click on Generate for i-frame service
3. Create order hash
Order hashing is a prerequisite for order creation, which acts as a validation layer of what your customer is purchasing and order amount. Order hash generation uses HMAC SHA256.
//Copy and paste this code in your Backend
let crypto = require("crypto");
function generateKashierOrderHash(order){
const mid = "MID-46-111";
const amount = order.amount;
const currency = order.currency;
const orderId = order.merchantOrderId;
const secret = "yourServiceSecretKey";
const path = `/?payment=${mid}.${orderId}.${amount}.${currency}`;
const hash = crypto.createHmac('sha256', secret).update(path).digest('hex');
return hash;}
//The Result Hash for /?payment=mid-0-1.99.20.EGP with secret 11111 should result 606a8a1307d64caf4e2e9bb724738f115a8972c27eccb2a8acd9194c357e4bec
//Copy and paste this code in your Backend
function generateKashierOrderHash($order){
$mid = "MID-46-111";
$amount = $order->amount;
$currency = $order->currency;
$orderId = $order->merchantOrderId;
$secret = "yourServiceSecretKey";
$path = "/?payment=".$mid.".".$orderId.".".$amount.".".$currency;
$hash = hash_hmac( 'sha256' , $path , $secret ,false);
return $hash; }
//The Result Hash for /?payment=mid-0-1.99.20.EGP with secret 11111 should result 606a8a1307d64caf4e2e9bb724738f115a8972c27eccb2a8acd9194c357e4bec
#Copy and paste this code in your Backend
import hmac
import hashlib
import binascii
def generateKashierOrderHash(order):
mid = "MID-46-111";
amount = order['amount']
currency = order['currency']
orderId = order['merchantOrderId']
path = '/?payment={}.{}.{}.{}'.format( mid, orderId, amount, currency )
path = bytes(path, 'utf-8')
secret = "yourServiceSecretKey"
secret = bytes(secret, 'utf-8')
return hmac.new(secret, path, hashlib.sha256).hexdigest()
#The Result Hash for /?payment=mid-0-1.99.20.EGP with secret 11111 should result 606a8a1307d64caf4e2e9bb724738f115a8972c27eccb2a8acd9194c357e4bec
//Copy and paste this code in your Backend
using System.Security.Cryptography;
public class Kashier
{
public static string create_hash()
{
string mid = "MID-46-111";
string amount = "20";
string currency = "EGP";
string orderId = "99";
string secret = "Secret Key";
string path = "/?payment=" + mid + "." + orderId + "." + amount + "." + currency;
string message;
string key;
key = secret;
message = path;
System.Text.ASCIIEncoding encoding = new System.Text.ASCIIEncoding();
byte[] keyByte = encoding.GetBytes(key);
byte[] messageBytes = encoding.GetBytes(message);
HMACSHA256 hmacmd256 = new HMACSHA256(keyByte);
byte[] hashmessage = hmacmd256.ComputeHash(messageBytes);
return ByteToString(hashmessage).ToLower();
}
public static string ByteToString(byte[] buff)
{
string sbinary = "";
for (int i = 0; i < buff.Length; i++)
{
sbinary += buff[i].ToString("X2"); // hex format
}
return (sbinary);
}
}
//The Result Hash for /?payment=mid-0-1.99.20.EGP with secret 11111 should result 606a8a1307d64caf4e2e9bb724738f115a8972c27eccb2a8acd9194c357e4bec
Parameters:
mid (string) |
Your Kashier Merchant ID |
amount (string) |
Order amount |
currency (string) |
Order currency (ISO: "EGP", "USD", "GBP" "EUR") |
orderId (string) |
Unique Order Identifier |
4. Add code snippet to your website
Fill in your order attributes, then add the following code snippet to your website.
src="https://test-iframe.kashier.io/js/kashier-checkout.js"
data-amount="ORDER-AMOUNT"
data-description="description"
data-hash="ORDER-HASH"
data-currency="ORDER-CURRENCY"
data-orderId="ORDER-ID"
data-merchantId="MID-91-106"
data-merchantRedirect="https://youwebsite/yourCallBack"
//data-merchantRedirect should be "URI encoded"
data-store = "XYZ"
data-type = "external"
data-display="en">
Parameters:
data-merchantId (string) |
Your Kashier Merchant ID |
data-amount (string) |
Order amount |
data-hash (string) |
Order hash generated in Step 3 |
data-currency (string) |
Order currency |
data-orderId (string) |
Unique Order Identifier |
data-merchantRedirect (string) |
data-merchantRedirect should be "URI encoded |
data-store (string) |
|
Hosted Payment Page
The Hosted Payment Page is a payment form with a redirection checkout experience. This form is built for merchants without platforms, interested in a fast and easy method integration.
1. Setup your Kashier account
Sign up to create a Kashier account
2. Generate an API Key
You can generate multiple i-frame API Keys as per your acceptance channels.
- Login to Kashier platform
- Navigate to Integrate now page
- Click on Generate for Hosted Payment Page service
3. Create order hash
Order hashing is a prerequisite for order creation, which acts as a validation layer of what your customer is purchasing and order amount. Order hash generation uses HMAC SHA256.
//Copy and paste this code in your Backend
let crypto = require("crypto");
function generateKashierOrderHash(order){
const mid = "MID-46-111";
const amount = order.amount;
const currency = order.currency;
const orderId = order.merchantOrderId;
const secret = "yourServiceSecretKey";
const path = `/?payment=${mid}.${orderId}.${amount}.${currency}`;
const hash = crypto.createHmac('sha256', secret).update(path).digest('hex');
return hash;}
//The Result Hash for /?payment=mid-0-1.99.20.EGP with secret 11111 should result 606a8a1307d64caf4e2e9bb724738f115a8972c27eccb2a8acd9194c357e4bec
//Copy and paste this code in your Backend
function generateKashierOrderHash($order){
$mid = "MID-46-111";
$amount = $order->amount;
$currency = $order->currency;
$orderId = $order->merchantOrderId;
$secret = "yourServiceSecretKey";
$path = "/?payment=.".$mid.".".$orderId.".".$amount.".".$currency;
$hash = hash_hmac( 'sha256' , $path , $secret ,false);
return $hash; }
//The Result Hash for /?payment=mid-0-1.99.20.EGP with secret 11111 should result 606a8a1307d64caf4e2e9bb724738f115a8972c27eccb2a8acd9194c357e4bec
#Copy and paste this code in your Backend
import hmac
import hashlib
import binascii
def generateKashierOrderHash(order):
mid = "MID-46-111";
amount = order['amount']
currency = order['currency']
orderId = order['merchantOrderId']
path = '/?payment={}.{}.{}.{}'.format( mid, orderId, amount, currency )
path = bytes(path, 'utf-8')
secret = "yourServiceSecretKey"
secret = bytes(secret, 'utf-8')
return hmac.new(secret, path, hashlib.sha256).hexdigest()
#The Result Hash for /?payment=mid-0-1.99.20.EGP with secret 11111 should result 606a8a1307d64caf4e2e9bb724738f115a8972c27eccb2a8acd9194c357e4bec
//Copy and paste this code in your Backend
using System.Security.Cryptography;
public class Kashier
{
public static string create_hash()
{
string mid = "MID-46-111";
string amount = "20";
string currency = "EGP";
string orderId = "99";
string secret = "Secret Key";
string path = "/?payment=" + mid + "." + orderId + "." + amount + "." + currency;
string message;
string key;
key = secret;
message = path;
System.Text.ASCIIEncoding encoding = new System.Text.ASCIIEncoding();
byte[] keyByte = encoding.GetBytes(key);
byte[] messageBytes = encoding.GetBytes(message);
HMACSHA256 hmacmd256 = new HMACSHA256(keyByte);
byte[] hashmessage = hmacmd256.ComputeHash(messageBytes);
return ByteToString(hashmessage).ToLower();
}
public static string ByteToString(byte[] buff)
{
string sbinary = "";
for (int i = 0; i < buff.Length; i++)
{
sbinary += buff[i].ToString("X2"); // hex format
}
return (sbinary);
}
}
//The Result Hash for /?payment=mid-0-1.99.20.EGP with secret 11111 should result 606a8a1307d64caf4e2e9bb724738f115a8972c27eccb2a8acd9194c357e4bec
Parameters:
mid (string) |
Your Kashier Merchant ID |
amount (string) |
Order amount |
currency (string) |
Order currency (ISO: "EGP", "USD", "GBP" "EUR") |
orderId (string) |
Unique Order Identifier |
4. Add code snippet to your website
Fill in your order attributes, then add the following code snippet to your website.
https://test-iframe.kashier.io/payment?mid=MID-46-111&orderId=ORDER-ID&amount=ORDER-AMOUNT¤cy=ORDER-CURRENCY&hash=ORDER-HASH&merchantRedirect=YOUR-SUCCESS-CALLBACK-URL
Parameters:
mid (string) |
Your Kashier Merchant ID |
amount (string) |
Order amount |
hash (string) |
Order hash generated in Step 3 |
currency (string) |
Order currency (ISO: "EGP", "USD", "GBP" "EUR") |
orderId (string) |
Unique Order Identifier |
merchantRedirect (string) |
Your callback URL - should be URI encoded |
Direct APIs
Getting Started
To be able to integrate directly with Kashier APIs, you'll need to go through the following steps,
1. Setup your Kashier account
Sign up to create a Kashier account
2. Generate an API Key
You can generate single/multiple customizable form service API Keys as per your acceptance channels.
- Login to Kashier platform
- Navigate to Integrate now page
- Click on Generate for Customizable form service
3. Create order hash
Order hashing is a prerequisite for order creation, which acts as a validation layer of what your customer is purchasing and order amount. Order hash generation uses HMAC SHA256.
const path = `/?payment=${mid}.${orderId}.${amount}.${currency}`;
const hash = crypto.createHmac('sha256', secret).update(path).digest('hex');
return hash;}
//The Result Hash for /?payment=mid-0-1.99.20.EGP with secret 11111 should result 606a8a1307d64caf4e2e9bb724738f115a8972c27eccb2a8acd9194c357e4bec
Parameters:
mid (string) |
Your Kashier Merchant ID |
amount (string) |
Order amount |
currency (string) |
Order currency (ISO: "EGP", "USD", "GBP" "EUR") |
orderId (string) |
Unique Order Identifier |
4. Environment Base URL
Please make sure to use one of the following Base URLs to integrate with our APIs.
Test mode https://test-iframe.kashier.io/
Live mode https://iframe.kashier.io/
Tokenization
Kashier provides you with 3 tokenization methods, where you can save card data as tokens based on your use case.
Multi-step Checkout form
(Temporary Card Token)
Expires in 10 minutes
Single-use
Save Card for Later
(Permanent Card Token)
Doesn't expire
Multi-use
Pay and Save card
(Permanent Card Token)
Doesn't expire
Multi-use
Multi-step Checkout form (Temporary Card Token)
Temporary Card Tokens are used for multi-step checkout forms, where you can save your customers card details across your checkout pages. Temporary card tokens expire in 10 minutes.
{
"hash":"dec4d0cae3e9206c42c0c9297337be1d2273385ea135a7fb76227b1a135b193a",
"merchantId":"MID-45-60",
"card_holder_name": "John Doe",
"card_number": "5111111111111118",
"ccv": "100",
"expiry_month": "05",
"expiry_year": "20",
"shopper_reference": "yourShopperId",
"tokenValidity":"temp"
}
Parameters:
hash (string) |
Order hash generated in Step 3 |
merchantId (string) |
Your Kashier Merchant ID |
card_holder_name (string) |
Cardholder name |
card_number (string) |
Card PAN number |
CVV (string) |
Card Verification Value/Code |
expiry_month (string) |
Card expiry month |
expiry_year (string) |
Card expiry year |
shopper_reference (string) |
Your customer ID/reference |
tokenValidity (string) |
Validity period of token (perm: permanent, temp: temporary) |
Response:
{
"status":200,
"body":{
"response":{
"cardToken":"11da69bc-a846-42ea-a9b2-b47a31a9f2d0",
"cardHolderName":"John Doe",
"maskedCard":"************1118",
"expiry_month":"05",
"expiry_year":"20"
},
"status":"SUCCESS"
}
}
Hash Failure Response:
{
"error":{
"cause":"invalid inputs"
},
"status":"FAILURE"
}
Save Card for Later (Permanent Card Token)
Permanent Card Tokens are mainly used for card-on file payments, where you can charge your customers multiple times without prompting them to enter their card details for each payment. Permanent card tokens don’t expire.
{
"hash":"dec4d0cae3e9206c42c0c9297337be1d2273385ea135a7fb76227b1a135b193a",
"merchantId":"MID-45-60",
"card_holder_name": "John Doe",
"card_number": "5111111111111118",
"ccv": "100",
"expiry_month": "06",
"expiry_year": "22",
"shopper_reference": "yourShopperId",
"tokenValidity":"perm"
}
Parameters:
hash (string) |
Order hash generated in Step 3 |
merchantId (string) |
Your Kashier Merchant ID |
card_holder_name (string) |
Cardholder name |
card_number (string) |
Card PAN number |
CVV (string) |
Card Verification Value/Code |
expiry_month (string) |
Card expiry month |
expiry_year (string) |
Card expiry year |
shopper_reference (string) |
Your customer ID/reference |
tokenValidity (string) |
Validity period of token (perm: permanent, temp: temporary) |
Response:
{
"status":200,
"body":{
"response":{
"cardToken":"11da69bc-a846-42ea-a9b2-b47a31a9f2d0",
"cardHolderName":"John Doe",
"maskedCard":"************1118",
"expiry_month":"05",
"expiry_year":"20"
},
"status":"SUCCESS"
}
}
Pay and Save card (Permanent Card Token)
This is the recommended payment integration method, as it enables you to pay and save card data for later use. This method tokenizes cards permanently.
{
"amount":"1",
"card_holder_name":"ibrahim",
"card_number":"5111111111111118",
"ccv":"100",
"currency":"EGP",
"display":"en",
"expiry_month":"06",
"expiry_year":"22",
"hash":"5c4607f52d695901c4e6e0c0e3cbc72a21c0e465495a3ade9030c1dbe39d40c9",
"merchantId":"MID-125-661",
"merchantRedirect":" ",
"orderId":"60663",
"saveCard":true,
"serviceName":"customizableForm",
"shopper_reference":"Meena_1"
}
Parameters:
amount (string) |
Your order amount |
card_holder_name (string) |
Cardholder name |
card_number (string) |
Card PAN number |
CVV (string) |
Card Verification Value/Code |
currency (string) |
Your order currency (ISO: “EGP”, “USD”, “GBP”, “EUR”) |
display (string) |
Should be “en” |
expiry_month (string) |
Card expiry month |
expiry_year (string) |
Card expiry year |
hash (string) |
Order hash generated in Step 3 |
merchantId (string) |
Your Kashier Merchant ID |
merchantRedirect (string) |
data-merchantRedirect should be "URI encoded |
orderId (string) |
Your order Identifier |
saveCard (string) |
Enum: should be “True” |
serviceName (string) |
Should be: “customizableForm |
shopper_reference (string) |
Your customer ID/reference |
Response:
{
"status":"SUCCESS",
"response":{
"sourceOfFunds":{
"provided":{
"card":{
"3DSecure":{
"processACSRedirectURL":"https://test-iframe.payformance.io/3ds/3a7d7683-a5bc-4bcc-b825-55e11e38ab18/d46b2c2b-6cd6-4baf-b7cc-623d915dc9e3/MID-125-661/mpgs/EGP",
"3DSecureId":"3DSecureId-d46b2c2b-6cd6-4baf-b7cc-623d915dc9e3"
},
"cardInfo":{
"cardDataToken":"282fe2f7-6189-42f6-bae6-ec4f99a66a55",
"ccvToken":"8cbc374c-d4b3-4306-a767-4dcc7f3a2428",
"cardHolderName":"ibrahim",
"cardHash":"b506253054c1bd027e272d6f9bf78cdb5087d4c7e56f5c2be7a45361ea274b1c",
"cardBrand":"Mastercard",
"maskedCard":"511111******1118"
},
"transaction":{ }
}
},
"type":"CARD"
},
"card":{
"cardInfo":{
"cardDataToken":"282fe2f7-6189-42f6-bae6-ec4f99a66a55",
"ccvToken":"8cbc374c-d4b3-4306-a767-4dcc7f3a2428",
"cardHolderName":"ibrahim",
"cardHash":"b506253054c1bd027e272d6f9bf78cdb5087d4c7e56f5c2be7a45361ea274b1c",
"cardBrand":"Mastercard",
"maskedCard":"511111******1118"
},
"order":{ },
"amount":1,
"currency":"EGP",
"result":"SUCCESS",
"merchant":{
"merchantRedirectUrl":" ?paymentStatus=SUCCESS&cardDataToken=&maskedCard=511111******1118&merchantOrderId=60663&orderId=3a7d7683-a5bc-4bcc-b825-55e11e38ab18&cardBrand=&orderReference=TEST-ORD-6432&transactionId=TX-1256611208&amount=1¤cy=EGP&signature=846e5a458b30b0bc5ad416394ea1f5bd13a85b62b0d7599e9b6115d11fbcad03"
}
},
"orderReference":"TEST-ORD-6432",
"orderId":"3a7d7683-a5bc-4bcc-b825-55e11e38ab18",
"transactionId":"TX-1256611208",
"merchantOrderId":"60663",
"creationDate":"2019-11-18T14:47:25.480Z",
"providerType":"mpgs",
"method":"card",
"apiKeyId":"5d837092be613d001c25f5b6"
},
"messages":{
"en":"Congratulations! Your payment was successful",
"ar":"تهانينا , لقد تم الدفع بنجاح"
}
}
List Saved Card Tokens
The ability to retrieve all shoppers saved/tokenized card tokens.
/tokens?mid=${MerchantId}&shopper_reference=${shopper_reference}&hash=${hash}
Accept Payments
Pay and Save Card
Permanently
Pay with Permanent Card
Token
Pay with Temporary Card
Token
Pay and Save card
This is the recommended payment integration method, as it enables you to pay and save card data for later use. This method tokenizes cards permanently.
{
"amount":"1",
"card_holder_name":"ibrahim",
"card_number":"5111111111111118",
"ccv":"100",
"currency":"EGP",
"display":"en",
"expiry_month":"06",
"expiry_year":"22",
"hash":"5c4607f52d695901c4e6e0c0e3cbc72a21c0e465495a3ade9030c1dbe39d40c9",
"merchantId":"MID-125-661",
"merchantRedirect":" ",
"orderId":"60663",
"saveCard":true,
"serviceName":"customizableForm",
"shopper_reference":"Meena_1"
}
Parameters:
amount (string) |
Your order amount |
card_holder_name (string) |
Cardholder name |
card_number (string) |
Card PAN number |
CVV (string) |
Card Verification Value/Code |
currency (string) |
Your order currency (ISO: “EGP”, “USD”, “GBP”, “EUR”) |
display (string) |
Should be “en” |
expiry_month (string) |
Card expiry month |
expiry_year (string) |
Card expiry year |
hash (string) |
Order hash generated in Step 3 |
merchantId (string) |
Your Kashier Merchant ID |
merchantRedirect (string) |
data-merchantRedirect should be "URI encoded |
orderId (string) |
Your order Identifier |
saveCard (string) |
Enum: should be “True” |
serviceName (string) |
Should be: “customizableForm |
shopper_reference (string) |
Your customer ID/reference |
Response:
{
"status":"SUCCESS",
"response":{
"sourceOfFunds":{
"provided":{
"card":{
"3DSecure":{
"processACSRedirectURL":"https://test-iframe.payformance.io/3ds/3a7d7683-a5bc-4bcc-b825-55e11e38ab18/d46b2c2b-6cd6-4baf-b7cc-623d915dc9e3/MID-125-661/mpgs/EGP",
"3DSecureId":"3DSecureId-d46b2c2b-6cd6-4baf-b7cc-623d915dc9e3"
},
"cardInfo":{
"cardDataToken":"282fe2f7-6189-42f6-bae6-ec4f99a66a55",
"ccvToken":"8cbc374c-d4b3-4306-a767-4dcc7f3a2428",
"cardHolderName":"ibrahim",
"cardHash":"b506253054c1bd027e272d6f9bf78cdb5087d4c7e56f5c2be7a45361ea274b1c",
"cardBrand":"Mastercard",
"maskedCard":"511111******1118"
},
"transaction":{ }
}
},
"type":"CARD"
},
"card":{
"cardInfo":{
"cardDataToken":"282fe2f7-6189-42f6-bae6-ec4f99a66a55",
"ccvToken":"8cbc374c-d4b3-4306-a767-4dcc7f3a2428",
"cardHolderName":"ibrahim",
"cardHash":"b506253054c1bd027e272d6f9bf78cdb5087d4c7e56f5c2be7a45361ea274b1c",
"cardBrand":"Mastercard",
"maskedCard":"511111******1118"
},
"order":{ },
"amount":1,
"currency":"EGP",
"result":"SUCCESS",
"merchant":{
"merchantRedirectUrl":" ?paymentStatus=SUCCESS&cardDataToken=&maskedCard=511111******1118&merchantOrderId=60663&orderId=3a7d7683-a5bc-4bcc-b825-55e11e38ab18&cardBrand=&orderReference=TEST-ORD-6432&transactionId=TX-1256611208&amount=1¤cy=EGP&signature=846e5a458b30b0bc5ad416394ea1f5bd13a85b62b0d7599e9b6115d11fbcad03"
}
},
"orderReference":"TEST-ORD-6432",
"orderId":"3a7d7683-a5bc-4bcc-b825-55e11e38ab18",
"transactionId":"TX-1256611208",
"merchantOrderId":"60663",
"creationDate":"2019-11-18T14:47:25.480Z",
"providerType":"mpgs",
"method":"card",
"apiKeyId":"5d837092be613d001c25f5b6"
},
"messages":{
"en":"Congratulations! Your payment was successful",
"ar":"تهانينا , لقد تم الدفع بنجاح"
}
}
Pay with Permanent Card Token
Kashier enables capturing payments using pre-saved permanent card tokens. Where you don’t have to require your customers to enter their card details before each transaction.
{
"merchantId":"MID-15-430",
"shopper_reference":"shopper",
"cardToken":"ab6fea2b-9c3c-47d2-a497-493b98c915c0",
"ccvToken":"62d3865e-4573-4acc-9cb4-21947862924f",
"amount":100,
"currency":"EGP",
"display":"en",
"hash":"92db97152a0fabbfd848e0c3bf884186c181161cfad78ac4e26c8c89f8b28626",
"orderId":"12334"
}
Parameters:
merchantId (string) |
Your Kashier Merchant ID |
shopper_reference (string) |
Your customer ID/reference |
cardToken (string) |
Your shopper’s card token |
amount (string) |
Your order amount |
currency (string) |
Your order currency (ISO: “EGP”, “USD”, “GBP”, “EUR”) |
display (string) |
Should be “en” |
hash (string) |
Order hash generated in Step 3 |
orderId (string) |
Your order Identifier |
serviceName (string) |
Should be: “customizableForm |
Response:
{
"response":{
"sourceOfFunds":{
"provided":{
"card":{
"3DSecure":{
"processACSRedirectURL":"http://localhost:8888/3ds"
},
"transaction":{
"recurring":true
}
}
},
"type":"CARD"
},
"card":{
"amount":“90”,
"currency":"EGP",
"result":"SUCCESS",
"merchant":{
"merchantRedirectUrl":"?paymentStatus=SUCCESS&cardDataToken=&maskedCard=&merchantOrderId=5dd78c07-6b8a-4b58-9032-6a4eefb5873b&orderId=e4d397b0-bdab-4f3f-9035-98e2848a0d7d&cardBrand=&orderReference=TEST-ORD-3829&transactionId=TX-3454934&amount=90¤cy=EGP"
}
},
"orderReference":"TEST-ORD-3829",
"orderId":"2f6450ea-1418-4975-822c-b5b4e0b79ed2",
"transactionId":"TX-3454934",
"merchantOrderId":"5dd78c07-6b8a-4b58-9032-6a4eefb5873b",
"creationDate":"2019-08-04T22:59:59.395Z",
"providerType":"mpgs",
"method":"card",
"refId":"56ce81d3-789a-4255-8864-beb16023ed94",
"merchantId":"MID-34-549"
},
"messages":{
"en":"Congratulations! Your payment was successful",
"ar":" , لقد تم الدفع بنجاح"
},
"status":"SUCCESS"
}
Hash Failure Response:
{
"status":"INVALID_REQUEST",
"error":{
"cause":"Invalid Token",
"explanation":"The used token is not valid"
}
}
Pay with Temporary Card Token
Kashier enables capturing payments using pre-saved temporary card tokens, which is recommended for multi-step checkout forms.
{
"merchantId":"MID-15-430",
"shopper_reference":"shopper",
"cardToken":"2d69ab51-0573-445e-bd43-b21f3a8a7ce3",
"amount":100,
"currency":"EGP",
"display":"en",
"hash":"d53ac4393b6bf5adc7a7db346570ffe22f84ae93810d9aec210c1a2e578f4fd5",
"orderId":"1234",
"serviceName":"customizableForm"
}
Parameters:
merchantId (string) |
Your Kashier Merchant ID |
shopper_reference (string) |
Your customer ID/reference |
cardToken (string) |
Your shopper’s card token |
ccvToken (string) |
Your shopper’s ccv Token |
amount (string) |
Your order amount |
currency (string) |
Your order currency (ISO: “EGP”, “USD”, “GBP”, “EUR”) |
display (string) |
Should be “en” |
hash (string) |
Order hash generated in Step 3 |
orderId (string) |
Your order Identifier |
serviceName (string) |
Should be: “customizableForm |
Response:
{
"response":{
"sourceOfFunds":{
"provided":{
"card":{
"3DSecure":{
"processACSRedirectURL":"http://localhost:8888/3ds"
},
"transaction":{
"recurring":true
}
}
},
"type":"CARD"
},
"card":{
"amount":“90”,
"currency":"EGP",
"result":"SUCCESS",
"merchant":{
"merchantRedirectUrl":"
?paymentStatus=SUCCESS&cardDataToken=&maskedCard=&merchantOrderId=5dd78c07-6b8a-4b58-9032-6a4eefb5873b&orderId=e4d397b0-bdab-4f3f-9035-98e2848a0d7d&cardBrand=&orderReference=TEST-ORD-3829&transactionId=TX-3454934&amount=90¤cy=EGP"
}
},
"orderReference":"TEST-ORD-3829",
"orderId":"2f6450ea-1418-4975-822c-b5b4e0b79ed2",
"transactionId":"TX-3454934",
"merchantOrderId":"5dd78c07-6b8a-4b58-9032-6a4eefb5873b",
"creationDate":"2019-08-04T22:59:59.395Z",
"providerType":"mpgs",
"method":"card",
"refId":"56ce81d3-789a-4255-8864-beb16023ed94",
"merchantId":"MID-34-549"
},
"messages":{
"en":"Congratulations! Your payment was successful",
"ar":" , لقد تم الدفع بنجاح"
},
"status":"SUCCESS"
}
Hash Failure Response:
{
"status":"INVALID_REQUEST",
"error":{
"cause":"Invalid Token",
"explanation":"The used token is not valid"
}
}
Woo-commerce
Kashier Woo-commerce Plugin allows you to accept payments through your e-commerce platform. This Plugin provides you with a seamless integration into your payment page directly on your website.
- Download Kashier Woocommerce Plugin
- Login to your Woocommerce Admin dashboard
- Go to Plugins section, then click Add new
- In the top-section, upload Kashier Woo-commerce Plugin by clicking on Upload plugin
- Click on Install Plugin and Activate Plugin
- Go to Woo-commerce section, then go to Settings
- Go to Payments section then select Kashier Plugin and enter your Test/Live API Key
- Click on Save changes and start accepting payments
WordPress
To integrate with Kashier for WordPress platform,
- Connect to your site’s server using FTP in WordPress
- Navigate to the wp-content folder within the WordPress installation for your website The location of your WordPress installation can differ with every hosting provider. Make sure that you know the location before you proceed
- Navigate to the /wp-content/plugins directory
- Navigate to wp-content. Inside this directory are the plugins and themes directories along with a few others
- Navigate to the plugins directory. It is inside this directory that all plugins reside
- Upload the plugin folder to the /wp-content/plugins directory on your web server
- Install & Activate the plugin, then go to your plugins directory and look for Kashier plugin
- Go to the plugin's settings and edit the Test/Live API Keys
PrestaShop
Kashier PrestaShop Plugin allows you to accept payments through your e-commerce platform. This Plugin provides you with a seamless integration into your payment page directly on your website.
- Download Kashier PrestaShop Plugin
- Login to your PrestaShop Admin dashboard
- Go to Modules and Services section, then upload Kashier PrestaShop Plugin
- Enable Plugin and click on configure
- Click on Test mode checkobx and enter your MID and Test API Key
- Click on save
Testing
You can use the following cards for testing your integration with Kashier.
Card type |
Card number |
Cardholder name |
MasterCard |
5123450000000008 (3D-Secure Enrolled) |
John Doe |
2223000000000007 (3D-Secure Enrolled) |
5111111111111118 |
2223000000000023 |
Visa |
4508750015741019 (3D-Secure Enrolled) |
4012000033330026 |
CSC/CVV response codes
CVV |
Response GW Code |
100 |
MATCH |
101 |
NOT_PROCESSED |
102 |
NO_MATCH |
Expiry date response codes
Expiry date |
Transaction Response Code |
06/22 |
APPROVED |
05/22 |
DECLINED |
04/27 |
EXPIRED_CARD |
08/28 |
TIMED_OUT |
01/37 |
ACQUIRER_SYSTEM_ERROR |
02/37 |
UNSPECIFIED_FAILURE |
05/37 |
UNKNOWN |